Project Name : Temperature Control with relay.
Project Description :
-
This is an ON OFF temperature Control.
-
The relay change over let you actuate an AC 220V device to control the temperature.
-
You can adjust the SetPoint to the range of 10 to 99 Degree C. with the left button (decrease by 1 C) or the right button (increase by 1 C)
Components :
-
1 - LCD 1602 I2C.
-
1 - Temperature sensor LM35.
-
2 - 12X12 Push Buttons.
-
2 - 10K Ohm Resistors.
-
2 - 330 ohm Resistors.
-
2 - Leds.
-
1 - one channel relay.
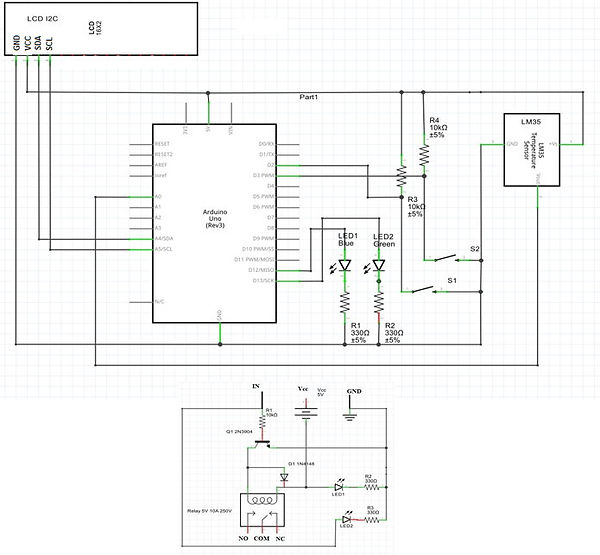
The code
======
/*
Testing LM36 thermometer
Signal leg connected to ANALOG Input AO
RElay IN leg connected to pin 9.
By Yohi Zucker
March 18th 2016
*/
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
LiquidCrystal_I2C lcd(0x27, 2, 1, 0, 4, 5, 6, 7, 3, POSITIVE);
const int button1Pin = 2; // pushbutton 1 pin
const int button2Pin = 3; // pushbutton 2 pin
float Temp;//Temperature variable
int Sensorpin = A0;//Read the temp sensor output
const int ledPin1 = 12; // LED pin1
const int ledPin2 = 13; // LED pin2
const int relayPin = 9; // Pin to actuate the relay
int SetTemp = 10;
void setup() {
pinMode(button1Pin, INPUT);
pinMode(button2Pin, INPUT);
pinMode(ledPin1, OUTPUT);
pinMode(ledPin2, OUTPUT);
pinMode(relayPin, OUTPUT);
Serial.begin(9600);
lcd.begin(16, 2);
lcd.backlight();
lcd.setCursor(2, 0);
lcd.print ("Temperature");
}
void loop() {
int button1State, button2State; // variables to hold the pushbutton states
button1State = digitalRead(button1Pin);
button2State = digitalRead(button2Pin);
if (button1State == LOW)
{
SetTemp = SetTemp - 1;
}
if (button2State == LOW)
{
SetTemp = SetTemp + 1;
}
if (SetTemp == 9)
{
SetTemp = 10;
}
if (SetTemp == 100)
{
SetTemp = 99;
}
lcd.setCursor(10, 1);
lcd.print("Set=");
lcd.setCursor(14, 1);
lcd.print(SetTemp);
//Serial.print( button1State);
//Serial.println( button2State);
delay(250);
lcd.setCursor(0, 1);
Temp = (5.0 * analogRead(Sensorpin) * 100.0) / 1024;
lcd.print(Temp);
lcd.print("C");
// Serial.print("Temperature= ");
//Serial.print(Temp);
//Serial.println("C");
//delay(1000);
if (Temp > SetTemp)
{
digitalWrite(ledPin2 , HIGH);
digitalWrite(ledPin1 , LOW);
digitalWrite(relayPin, HIGH);
}
else
{
digitalWrite(ledPin2 , LOW);
digitalWrite(ledPin1 , HIGH);
digitalWrite(relayPin, LOW);
}
}